How to Automatically Delete Videos from Your YouTube Watch Later Playlist
YouTube is a great platform to save videos for watching later, but those Watch Later lists can quickly become cluttered with old videos you no longer want to keep. Manually deleting them can be time-consuming. In this guide, I’ll walk you through a simple way to automate the process of removing videos from your Watch Later playlist using a JavaScript script.
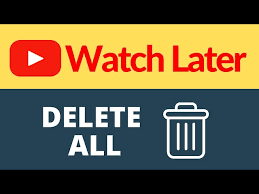
Step-by-Step Guide to Deleting Videos from Watch Later on YouTube
Follow these steps to quickly remove multiple videos from your YouTube Watch Later playlist.
1. Open the YouTube Watch Later Playlist
Navigate to YouTube's Watch Later playlist in your browser. You should see all the videos you've saved to watch later.
2. Open Developer Tools
Press F12
(or right-click on the page and select Inspect) to open your browser’s Developer Tools. You’ll be using the Console tab to run the JavaScript code.
3. Copy and Paste the Code Below
In the Console tab, paste the following script to automate the deletion of videos:
;(function () {
let count = 0
const maxVideosToDelete = 5
function deleteFirstVideo() {
const videoItems = document.querySelectorAll('ytd-playlist-video-renderer')
if (videoItems.length === 0 || count >= maxVideosToDelete) {
console.log(`Deleted ${count} videos.`)
return
}
const menuButton = videoItems[0].querySelector(
'ytd-menu-renderer yt-icon-button',
)
if (menuButton) {
menuButton.click()
setTimeout(() => {
const removeButton = [
...document.querySelectorAll('tp-yt-paper-item'),
].find((item) =>
item.innerText.toLowerCase().includes('remove from watch later'),
)
if (removeButton) {
removeButton.click()
count++
console.log(`Deleted ${count} video(s).`)
setTimeout(deleteFirstVideo, 1000)
} else {
console.log('Could not find remove button. Retrying...')
setTimeout(deleteFirstVideo, 1000)
}
}, 500)
} else {
console.log('Menu button not found. Retrying...')
setTimeout(deleteFirstVideo, 1000)
}
}
deleteFirstVideo()
})()
4. Run the Script
Once you’ve pasted the code, press Enter. The script will automatically start deleting the first few videos from your Watch Later playlist (up to 5 videos by default). If you want to delete more than 5 videos, you can adjust the value of maxVideosToDelete
in the script. The process will run in the background, and you can see updates in the Console like "Deleted X video(s)" for each removed video.
5. Watch the Script in Action
As the script runs, you will notice that the videos are being removed one by one from your Watch Later playlist. Each time a video is deleted, the console will log a message confirming the deletion. If the script encounters any issues (like failing to find the "Remove from Watch Later" button), it will retry after a brief delay.
Pros and Cons of Using This Automation Script
Pros:
- Time-saving: Automates the process of deleting videos from the Watch Later playlist, which would otherwise be tedious and manual.
- Customizable: You can adjust the
maxVideosToDelete
value to delete as many or as few videos as you need. - Simple to use: No advanced coding knowledge is required—just copy and paste the code into the browser's Developer Tools.
- Efficient for batch deletions: Removes multiple videos quickly, with each deletion happening automatically.
Cons:
- Manual execution: Although the process is automated, you still need to open Developer Tools and manually run the script.
- YouTube layout changes: YouTube’s UI may change over time, which could break the script. If that happens, you’ll need to update the script selectors.
Things to Keep in Mind
- Test carefully: Although the script is safe, it’s always good practice to test it on a few videos before running it for large batches.
- Modifying the script: You can easily change the
maxVideosToDelete
value to delete more or fewer videos at once. By default, it deletes 5 videos. - YouTube layout updates: YouTube frequently updates its interface, which may affect how the script interacts with the site. If the script stops working, inspect the elements using the Developer Tools and update the relevant selectors in the code.
- Browser compatibility: This script is designed for Chrome and Firefox. It should work similarly in other browsers, but you may notice slight differences in behavior.